Web API にBearer認証を追加する
(参考)ASP.NET Core の認証の概要
前提環境
Visual Studio2019
Windows10
Web API プロジェクト
Swagger
開発
認証機能の登録
認証の設定を追加。
using Microsoft.AspNetCore.Authentication.JwtBearer; // 追加 ・・・ public void ConfigureServices(IServiceCollection services) { services.AddRazorPages(); // ↓ 追加 services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme).AddJwtBearer(options => { options.TokenValidationParameters = new TokenValidationParameters { ValidateIssuer = true, ValidateAudience = true, ValidateLifetime = true, ValidateIssuerSigningKey = true, ValidIssuer = TokenController.JwtIssure, ValidAudience = TokenController.JwtIssure, IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(TokenController.JwtKey)) }; }); // ↑ 追加 ・・・ public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { ・・・ app.UseAuthentication(); // 追加 app.UseAuthorization(); ・・・ app.UseEndpoints(endpoints => { endpoints.MapRazorPages(); endpoints.MapControllers(); // 追加 });
エラー(赤い波線)があったら、マウスカーソルを合わせると「考えられる修正内容を表示する」からNuGetで「Microsoft.AspNetCore.Authentication.JwtBearer」をインストールすればよいことがわかる。
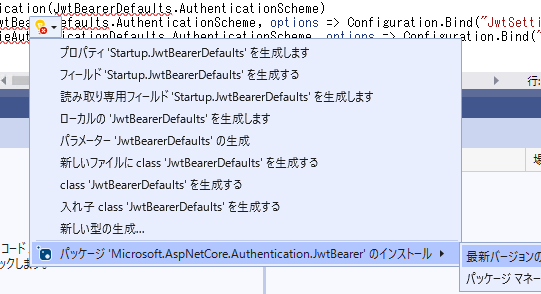
コントローラ追加
トークンコントローラーを作成。
using System; using System.ComponentModel.DataAnnotations; using System.IdentityModel.Tokens.Jwt; using System.Text; using Microsoft.AspNetCore.Authorization; using Microsoft.AspNetCore.Mvc; using Microsoft.IdentityModel.Tokens; using Newtonsoft.Json; namespace WebApplication2.Controllers { [Route("api/[controller]")] [ApiController] public class TokenController : ControllerBase { public static string JwtKey = "1234567890123456789012345678901234567890"; public static string JwtIssure = "JwtIssure"; [AllowAnonymous] [HttpPost] public IActionResult Login([FromBody] LoginModel request) { // 認証 if (request.user == "111" && request.pass == "111") { return Ok(new { Token = BuildToken() }); } else { return BadRequest(); } } private string BuildToken() { var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(JwtKey)); var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256); var token = new JwtSecurityToken( issuer: JwtIssure, audience: JwtIssure, expires: DateTime.Now.AddMinutes(30), signingCredentials: creds ); return new JwtSecurityTokenHandler().WriteToken(token); } public class LoginModel { [Required] public string user { get; set; } [Required] public string pass { get; set; } } } }
Swaggerの設定
Swaggerを入れている場合は、Bearerトークンを指定できるようにする。
//services.AddSwaggerGen(); services.AddSwaggerGen(c => { c.SwaggerDoc("v1", new OpenApiInfo { Title = "TodoApi", Version = "v1" }); c.AddSecurityDefinition("Bearer", new OpenApiSecurityScheme { Description = @"Example: 'Bearer 12345abcdef'", Name = "Authorization", In = ParameterLocation.Header, Type = SecuritySchemeType.ApiKey, Scheme = "Bearer" }); c.AddSecurityRequirement(new OpenApiSecurityRequirement() { { new OpenApiSecurityScheme { Reference = new OpenApiReference { Type = ReferenceType.SecurityScheme, Id = "Bearer" }, Scheme = "oauth2", Name = "Bearer", In = ParameterLocation.Header, }, new List<string>() } }); });
Authorizeの鍵マークが表示される。
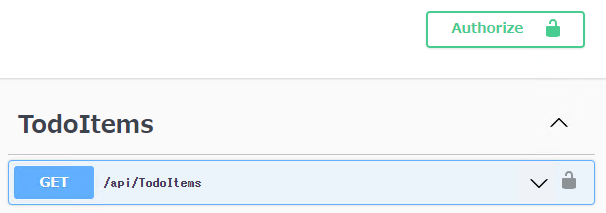
実行
認証しないで「https://localhost:5001/api/TodoItems」にアクセスするとエラーになることを確認。
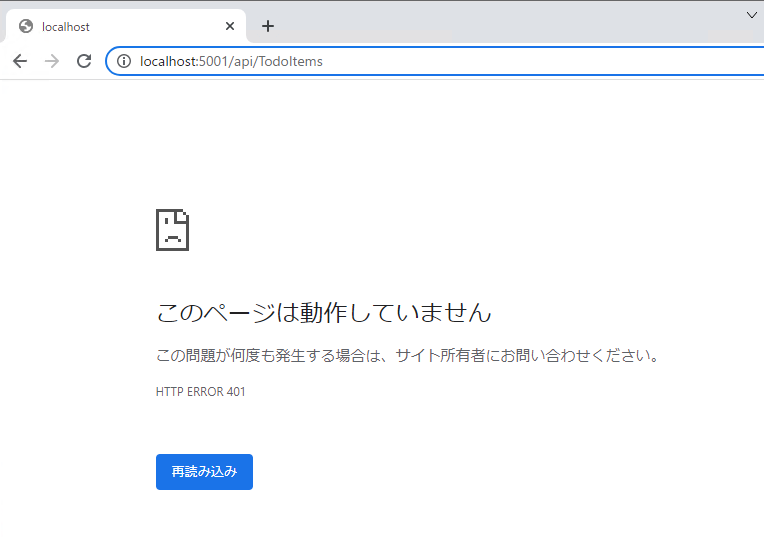
Swaggerで確認。HTTPエラー401となる。
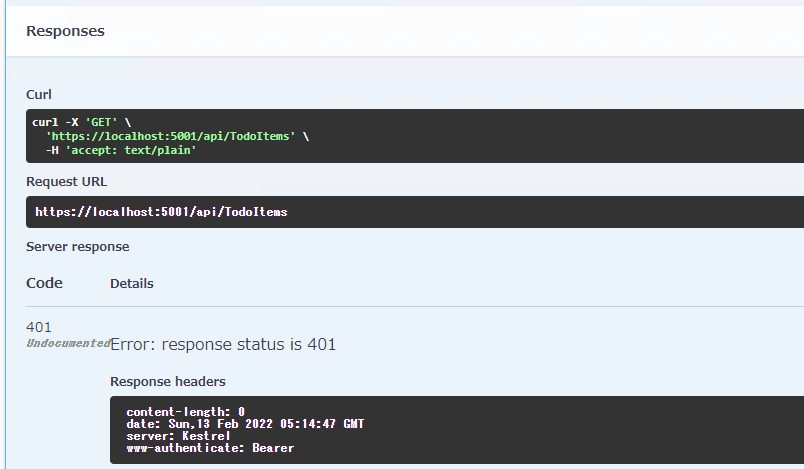
ログインしてトークンを取得。

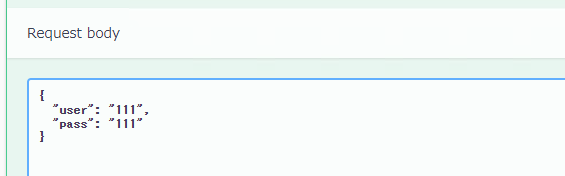
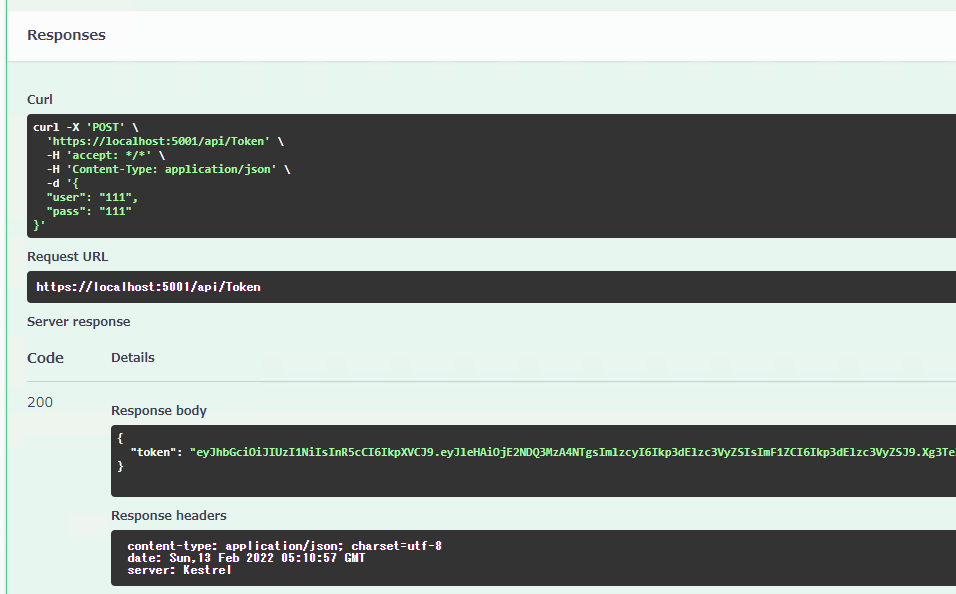
鍵マークをクリックし、Valueに「bearer ○○○○」と入力、「Authorize」→「Close」。
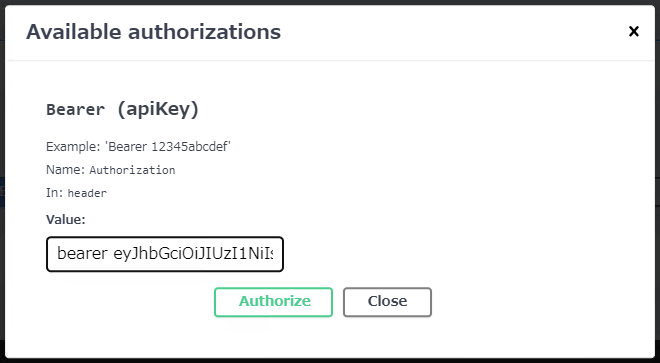
成功。
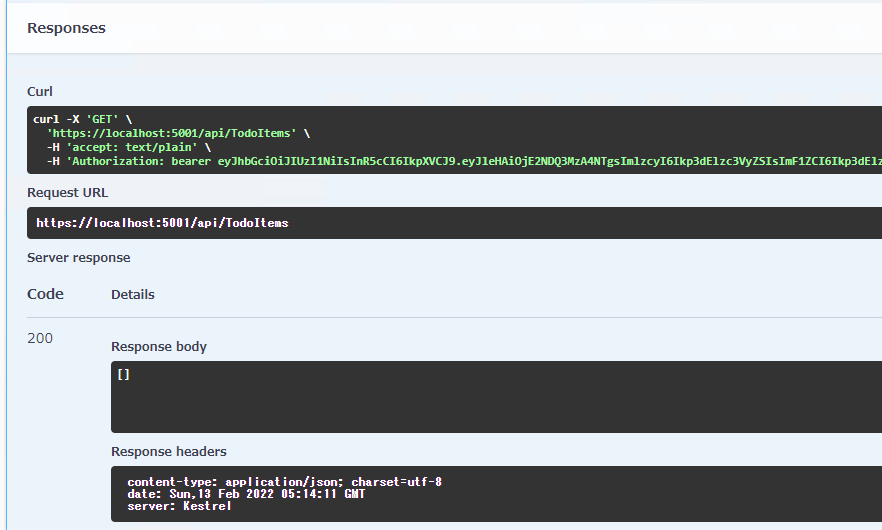
コメント